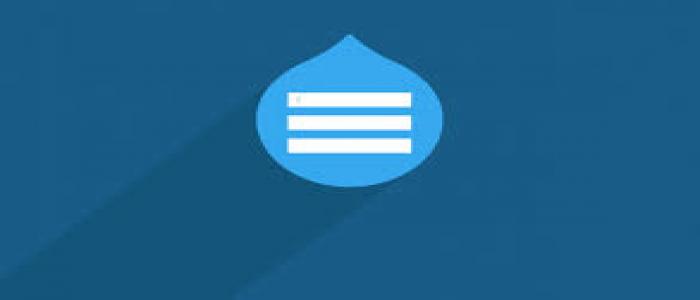
در این اموزش به فرم ها در دروپال ۸ میپردازیم
آموزش ماژول نویسی دروپال 8 قسمت 4
آموزش ماژول نویسی دروپال 8 قسمت 3
آموزش ماژول نویسی دروپال 8 قسمت 2
آموزش ماژول نویسی دروپال 8 قسمت 1
فرم ها در دروپال 8
ما در این مرحله ماژولی را که نوشه بودیم گسترش میدهیم
ابتدا در روت اصلی ماژول یه پوشه جدید به اسم Form ایجاد میکنیم و در پوشه ایجاد شده فایل به نام CustomForm.php ایجاد میکنیم و در فایل ایجاد شده کد ها را به صورت زیر مینویسیم
<?<?php
namespace Drupal\custom\form;
use Drupal\Core\Form\FormBase;
use Drupal\Core\Form\FormInterface;
use Drupal\Core\Form\FormStateInterface;
class CustomForm extends FormBase {
}
در اینجا بک namespace برای فرم خودمون تعریف میکنم
و مقادیر FormInterface و FormStateInterface برای این شاخه use میکنیم
در ادامه باید برای این فرم یک form id ایجاد کینم
/**
* Returns a unique string identifying the form.
*
* The returned ID should be a unique string that can be a valid PHP function
* name, since it's used in hook implementation names such as
* hook_form_FORM_ID_alter().
*
* @return string
* The unique string identifying the form.
*/
public function getFormId() {
return 'my_custom_form';
}
بعد میتوانیم فیلد های خودمون رابه صورت زیر ایجاد کینم
/**
* Form constructor.
*
* @param array $form
* An associative array containing the structure of the form.
* @param \Drupal\Core\Form\FormStateInterface $form_state
* The current state of the form.
*
* @return array
* The form structure.
*/
public function buildForm(array $form, FormStateInterface $form_state) {
$form['description'] = [
'#type' => 'item',
'#markup' => $this->t('Please enter the title and accept the terms of use of the site.'),
];
$form['title'] = [
'#type' => 'textfield',
'#title' => $this->t('Title'),
'#description' => $this->t('Enter the title of the book. Note that the title must be at least 10 characters in length.'),
'#required' => TRUE,
];
$form['accept'] = array(
'#type' => 'checkbox',
'#title' => $this
->t('I accept the terms of use of the site'),
'#description' => $this->t('Please read and accept the terms of use'),
);
$form['actions'] = [
'#type' => 'actions',
];
$form['actions']['submit'] = [
'#type' => 'submit',
'#value' => $this->t('Submit'),
];
return $form;
}
برای فرم میتوانیم یک ولیدت ایجاد کنیم
public function validateForm(array &$form, FormStateInterface $form_state) {
parent::validateForm($form, $form_state);
$title = $form_state->getValue('title');
$accept = $form_state->getValue('accept');
if (strlen($title) < 10) {
// Set an error for the form element with a key of "title".
$form_state->setErrorByName('title', $this->t('The title must be at least 10 characters long.'));
}
if (empty($accept)){
// Set an error for the form element with a key of "accept".
$form_state->setErrorByName('accept', $this->t('You must accept the terms of use to continue'));
}
}
و در انتها مقدار submit را تعریف میکنم
public function submitForm(array &$form, FormStateInterface $form_state) {
drupal_set_message($form_state->getValue('title'));
return;
}
در نهایت ماژول به صورت زیر خواهد بود
<?php
namespace Drupal\custom\form;
use Drupal\Core\Form\FormBase;
use Drupal\Core\Form\FormInterface;
use Drupal\Core\Form\FormStateInterface;
class CustomForm extends FormBase {
/**
* Returns a unique string identifying the form.
*
* The returned ID should be a unique string that can be a valid PHP function
* name, since it's used in hook implementation names such as
* hook_form_FORM_ID_alter().
*
* @return string
* The unique string identifying the form.
*/
public function getFormId() {
return 'my_custom_form';
}
/**
* Form constructor.
*
* @param array $form
* An associative array containing the structure of the form.
* @param \Drupal\Core\Form\FormStateInterface $form_state
* The current state of the form.
*
* @return array
* The form structure.
*/
public function buildForm(array $form, FormStateInterface $form_state) {
$form['description'] = [
'#type' => 'item',
'#markup' => $this->t('Please enter the title and accept the terms of use of the site.'),
];
$form['title'] = [
'#type' => 'textfield',
'#title' => $this->t('Title'),
'#description' => $this->t('Enter the title of the book. Note that the title must be at least 10 characters in length.'),
'#required' => TRUE,
];
$form['accept'] = array(
'#type' => 'checkbox',
'#title' => $this
->t('I accept the terms of use of the site'),
'#description' => $this->t('Please read and accept the terms of use'),
);
$form['actions'] = [
'#type' => 'actions',
];
$form['actions']['submit'] = [
'#type' => 'submit',
'#value' => $this->t('Submit'),
];
return $form;
}
public function validateForm(array &$form, FormStateInterface $form_state) {
parent::validateForm($form, $form_state);
$title = $form_state->getValue('title');
$accept = $form_state->getValue('accept');
if (strlen($title) < 10) {
// Set an error for the form element with a key of "title".
$form_state->setErrorByName('title', $this->t('The title must be at least 10 characters long.'));
}
if (empty($accept)){
// Set an error for the form element with a key of "accept".
$form_state->setErrorByName('accept', $this->t('You must accept the terms of use to continue'));
}
}
public function submitForm(array &$form, FormStateInterface $form_state) {
drupal_set_message($form_state->getValue('title'));
return;
}
}
میتوانیم یک route جدید برای فرم تعرف کنیم \
mycustom.content:
path: '/hello'
defaults:
_controller: 'Drupal\mycustom\Controller\MycustomController::content'
_title: 'Hello world'
requirements:
_permission: 'my custom permisstion'
mycustom.form:
path: '/csfrom'
defaults:
_form: 'Drupal\mycustom\Form\MycustomForm'
_title: 'Hello world'
requirements:
_permission: 'my custom permisstion'
افزودن دیدگاه جدید